Have you ever thought about style management? Styling is one of many processes during the web building process. As the development grows, its stylesheets increase in size and complexity, making them harder to maintain as time passes. That’s where a CSS pre-processor comes in handy.
What is SASS/SCSS?
SASS (Syntactically Awesome Stylesheet) / SCSS (Sassy Cascading Style Sheets) are CSS pre-processors that add special features such as variables, mixing, nesting selector, and inheritance selector. While their functions remain the same, the main difference between SASS and SCSS is their syntax. For example, SASS does not require curly brackets.
Here are four significant advantages:
- Variables -> to store information that is to be re-used later
- Nesting -> to allow you to nest CSS selectors in the same way as HTML
- Mixin -> to allow you to create CSS code that can be re-used
- Interpolation -> a mixin function that can pass expressions
Demonstration
Let’s jump into the codes. This article will provide examples of three types of extensions for us to learn the differences between SASS, SCSS, and CSS. Let’s use ReactJs for our demonstration to make it simple and easy.
Open the terminal and complete these commands to spin up a new react project with SASS/SCSS ready to use.
- npx create-react-app react-SASS
- cd react-sass
- npm install node-sass -save
- npm start
In App.js
Please note that you can either use SASS or SCSS. First, let’s demonstrate how to use SASS as our style extension.
import './App.sass';
function App() {
return (
<div className="App">
<div className="purple">
<p>Purple</p>
</div>
<div className="divider"></div>
<div className="black">
<p>Black</p>
</div>
</div>
);
}
export default App;
Let’s make our SASS file by creating a file called App.sass.
//variable example
$i-want-turquoise: turquoise
$i-want-black: black
$i-want-purple: purple
//mixin example
@mixin div-style
width: 100%
height: 100%
align-items: center
display: flex
justify-content: center
@mixin p-style
padding: 20px
font-size: 60px
font-weight: bold
text-align: center
//interpolation example
@mixin div-theme($name, $bg-color, $color)
#{$name}
background-color: #{$bg-color}
color: #{$color}
//the line below is an example of how to call the interpolation method
@include div-theme(".black", $i-want-black, $i-want-purple)
@include div-theme(".purple", $i-want-purple, $i-want-black)
.App
display: flex
height: 100vh
.purple
//the line below is an example of how to call the existing mixin
@include div-style
p //this p tag is placed inside the called class, which represents the Nesting method
//the line below is an example of how to call the existing mixin
@include p-style
//the line below is an example of how to call the variables that stated earlier
.divider
width: 5%
background-color: $i-want-turquoise
.black
//the line below is an example of how to call the existing mixin
@include div-style
p //this p tag is placed inside the called class, which represents the Nesting method
//the line below is an example of how to call the existing mixin
@include p-style
That is how we use SASS. Now, let’s build the SCSS extension by creating a file called App.scss.
//variable example
$i-want-turquoise: turquoise;
$i-want-black: black;
$i-want-purple: purple;
//mixin example
@mixin div-style{
width: 100%;
height: 100%;
align-items: center;
display: flex;
justify-content: center;
}
@mixin p-style {
padding: 20px;
font-size: 60px;
font-weight: bold;
text-align: center;
}
//interpolation example
@mixin div-theme($name, $bg-color, $color) {
#{$name} {
background-color: #{$bg-color};
color: #{$color};
}
}
//the line below is an example of how to call the interpolation method
@include div-theme(".black", $i-want-black, $i-want-purple);
@include div-theme(".purple", $i-want-purple, $i-want-black);
.App {
display: flex;
height: 100vh;
}
.purple{
//the line below is an example of how to call the existing mixin
@include div-style;
p{ //this p tag placed inside the called class, which represents the Nesting method
//the line below is an example of how to call the existing mixin
@include p-style;
}
}
//the line below is an example of how to call the variables that stated earlier
.divider{
width: 5%;
background-color: $i-want-turquoise;
}
.black{
//the line below is an example of how to call the existing mixin
@include div-style;
p{ //this p tag placed inside the called class, which represents the Nesting method
//the line below is an example of how to call the existing mixin
@include p-style;
}
}
Suppose you compare the two style pre-processor files. The syntax is different, but their function remains the same, generating this page.
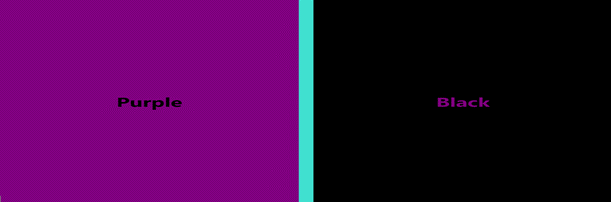
As a comparison, if we use CSS as our stylesheet, the code below will be the result, generating many duplications of properties with the same value.
.black {
background-color: black;
color: purple;
}
.purple {
background-color: purple;
color: black;
}
.App {
display: flex;
height: 100vh;
}
.purple {
width: 100%;
height: 100%;
align-items: center;
display: flex;
justify-content: center;
}
.purple p {
padding: 20px;
font-size: 60px;
font-weight: bold
text-align: center;
}
.divider {
width: 5%;
background-color: turquoise;
}
.black {
width: 100%;
height: 100%;
align-items: center;
display: flex;
justify-content: center;
}
.black p {
padding: 20px;
font-size: 60px;
font-weight: bold;
text-align: center;
}
Conclusion
Using SASS/SCSS style pre-processors, we can quickly write code while preventing the duplication of called properties with the same value.
This article has covered the primary or essential features of using variables, nesting, mixin, and interpolation features. There are many uses for SASS/SCSS. To find out more, check out their official page here https://sass-lang.com/documentation.
Author: Danny Gandasasmita, Programmer