If you have ever developed an Android application and struggled to create a UI using XML files, maybe it’s time for you to switch to using Jetpack Compose and develop your UI using Kotlin functions.
What is Jetpack Compose?
Jetpack Compose is Android’s modern toolkit for building native UI. With Jetpack Compose you can simplify your code when building a UI. If you have ever developed an application using Flutter / SwiftUI then you will be familiar with the structure that will be used in Jetpack Compose because all three languages implement declarative programming.
The @Composable annotation is used to identify composable functions. This annotation is required for composable functions because it informs the compiler that this function adds UI to the View Hierarchy. Composable functions can call standard functions, but Composable can only be called by other Composable.
Why is Jetpack Compose better than traditional UI development?
- Less Code
Less code means less testing, less bug-hunting, and more time for developers to concentrate on the task at hand. Writing less code also reduces the amount of code a developer must read, comprehend, review, and maintain.
- Intuitive
With declarative programming, to build UI you only need to describe the properties, so it becomes more understandable to write and read the code and of course more easily maintainable in the future.
- Faster
All your currently written code is compatible with Compose. Both Views and Compose can call each other’s code. Compose supports most libraries from Android Jetpack, so you can start to implement Compose in your project without worrying about unsupported libraries. Using the full Android Studio support, you get to iterate and ship code faster.
- Powerful
Compose comes with support for Material Design, Dark themes, and animations to bring movement and life to your apps. As material Design is separate from the foundation, it allows developers to build their own design systems.
What are the basic features of Jetpack Compose?
Composable
Add the @Composable annotation to a function to make it composable. To test this, create a Greeting function that receives a name and uses it to configure the text element.
Preview
The @Preview annotation in Android Studio allows you to preview your composable functions without having to build and install the app on an Android device or emulator. The annotation can only be applied to a composable function that does not accept parameters. As a result, you can’t directly preview the Greeting function. Instead, create a second function called PreviewGreeting that calls Greeting with a suitable parameter. Add @Preview annotation before @Composable.
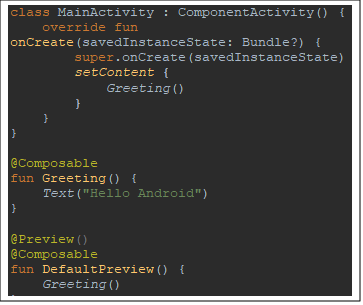
Layout Basic
@Composable
fun Greeting() {
Text("Hello Android")
Text("How are you?")
You need to wrap the components into a container like a Column, Row, or Box to arrange the components. Otherwise, the components will stack on top of each other, making the UI messy.
So, we use Column to place arrange items vertically in the same way as LinearLayout with vertical orientation
@Composable
fun Greeting() {
Column{
Text("Hello Android")
Text("How are you?")
}
}
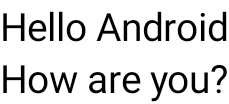
Column
The column function can stack UI elements vertically. This function will stack all the sequentially in a vertical manner with no spacing between them. It is annotated with Column().
Row
The row function stacks UI elements horizontally. Similar to Column(), this function stacks the children one after the other horizontally with no spacing between them. It is annotated with Row().
Box
A widget that is used to position elements one on top of the other. It positions the children relative to its edges. The stack is used to draw the children which will overlap in the order that they are specified. It is annotated with Box().
Spacer
It is used to give spacing between two views. We can specify the height and width of the box. It is an empty box that is used to give spacing between the views. It is annotated with Spacer().
Vertical Scroll
If the UI components inside the app do not fit the height of the screen then we have to use the scrolling view. With the help of a vertical scroller, we can provide a vertically scrolling behavior to our view. The contents inside the vertical scroller will be clipped to the bounds of the vertical scroller. It is annotated with VerticalScroll().
Padding
The padding function is used to provide extra white around a specific view. It is simply annotated with Padding().
Lazy List
This composable is similar to a recycler view in Android’s view system. It is annotated with LazyColumn()..
Conclusion
At Mitrais our developers have simplified and accelerated their UI development on Android, bringing their apps to life with less code, powerful tools, and intuitive Kotlin APIs. By making Android UI app building faster, easier, and more maintainable, they can deliver increased value to our customers.
You can explore more about Compose Layout here: Link
Author: Muhammad Adhi Faizal, Software Engineer Analyst Programmer