When building code and projects for your web application, following common patterns help make code more accessible and easier to manage.
Software design patterns in PHP are reusable solutions to common programming problems. The patterns show relationships and interactions between classes or objects. Patterns are object-oriented programming, independent language strategies that solve everyday problems during the software development process and enable more flexible, reusable, and maintainable code. Providing well-tested, proven development/design paradigms can hasten the development process.
Design Patterns
A few of the design patterns include:
- Abstract Factory
- Builder
- Factory Method
- Prototype
- Singleton
- Adapter
- Bridge
- Composite
Builder Pattern Example
In the PHP world, the Builder Pattern is well-known and used in many PHP frameworks like CodeIgniter and Laravel.
The PHP Builder Design Pattern is a creational design pattern that could build specific class objects with possibilities to set properties and objects dynamically. It is instrumental when creating an object with many possible configuration options.
In the Builder Pattern, a director and a builder work together to build an object. The director controls the building and specifies the parts and variations of an object. The builder knows how to assemble the given object specification.
The Builder Pattern creates complex systems with simple objects and a step-by-step approach. Software patterns separate complex objects from their representations so that the same construction process can make different representations. The Builder Pattern is a way to untangle how to create an object from the object class itself.
Here is an example of PHP code programming with an already implemented builder design pattern.
For this example, we will use Visual Studio code and XAMPP for executing the PHP application in Windows OS. You will need basic PHP knowledge for implementing functions and OOP.
CAR Class
<?php
class Car {
private $brand;
private $machineCapacity;
private $machineType;
private $mileage;
public function __construct($brand, $machineCapacity,$machineType, $mileage){
$this->brand = $brand;
$this->machineCapacity = $machineCapacity;
$this->machineType = $machineType;
$this->mileage = $mileage;
}
public function getMileAge(){
return $this->mileage;
}
public function getmachineCapacity(){
return $this->machineCapacity;
}
public function getMachineType(){
return $this->machineType;
}
public function getBrand(){
return $this->brand;
}
public function setBrand($brand){
if(null !== $brand){
$this->brand = $brand;
return "Brand Saved Succesfully";
}else{
return "You need to enter the brand name";
}
}
public function addMileage($mileage){
$this->mileage += $mileage;
}
}
CarBuilderClass
<?php
include('Car.php');
class CarBuilder {
private $brand = 'Unknown';
private $machineCapacity = 'Unknown';
private $machineType = 'Unknown';
private $mileage = 0 ;
public function setBrand($brand){
$this->brand = $brand;
return $this;
}
public function setMachineCapacity($capacity){
$this->machineCapacity = $capacity;
return $this;
}
public function setMachineType($type){
$this->machineType = $type;
return $this;
}
public function setmileAge($mile){
$this->mileage = $mile;
return $this;
}
public function build():Car {
$car = new Car($this->brand,$this->machineCapacity,$this->machineType,$this->mileage);
return $car;
}
}
?>
The Index File
<?php
include('Carbuilder.php');
$cars = array();
$avansa = (new CarBuilder())
->setBrand('Toyota')
->setMachineType('Petrol')
->setMileage(8000)
->build();
array_push($cars,$avansa);
$fuso = (new CarBuilder())
->setBrand('Mithsubishi')
->setMachineType('Diesel')
->setMachineCapacity(2000)
->build();
array_push($cars,$fuso);
?>
<table>
<thead >
<th style="border : 1px solid black; padding : 4px;" >Brand</th>
<th style="border : 1px solid black; padding : 4px;" >Machine Capacity</th>
<th style="border : 1px solid black; padding : 4px;" >Machine Type</th>
<th style="border : 1px solid black; padding : 4px;">Mileage</th>
</thead>
<tbody>
<?php foreach($cars as $item){ ?>
<tr >
<td style="border : 1px solid black; padding : 4px;" > <?php echo $item->getBrand(); ?></td>
<td style="border : 1px solid black; padding : 4px;" > <?php echo $item->getMachineCapacity(); ?> </td>
<td style="border : 1px solid black; padding : 4px;"> <?php echo $item->getMachineType(); ?> </td>
<td style="border : 1px solid black; padding : 4px;"> <?php echo $item->getMileage(); ?> </td>
</tr>
<?php } ?>
</tbody>
</table>
Output
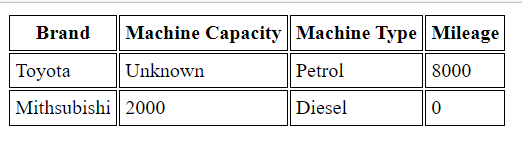
You may have used the Builder Pattern if you are familiar with the Laravel or CodeIgniter framework, especially when using the query builder. However, you may have used it without knowing what happened in the background process.
Reference
https://sourcemaking.com/design_patterns
https://www.logique.co.id/blog/2021/02/04/software-design-pattern/
https://sourcemaking.com/design_patterns/builder/php/1
https://www.kursuswebsite.org/cara-menggunakan-builder-pattern-di-php/
Author: Wayan Widya Artana, Analyst Programmer